Chapter #7 – Functions
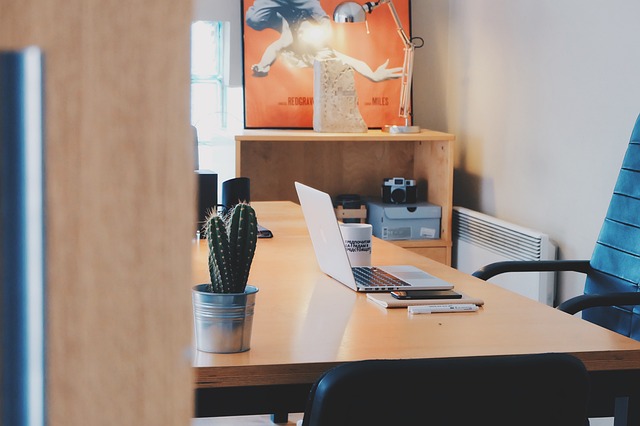
Chapter #7 – Q #6:
Differentiate between following with the help of an example:
a) Argument and Parameter
Parameters: These are the variables defined in a function declaration. They act as placeholders for the values that will be passed to the function when it is called.
Arguments: These are the actual values that are passed to the function when it is invoked.
Example:
def greet(name): # 'name' is a parameter
print(f"Hello, {name}!")
greet("Alice") # "Alice" is an argument
greet("Bob") # "Bob" is another argument
b) Global and Local variable.
Local Variables: These are variables defined within a function or block and can only be accessed within that specific function or block.
Global Variables: These are variables defined outside of any function and can be accessed from any part of the code, including inside functions.
Example:
# Global variable
counter = 0
def increment():
global counter # Declare counter as global to modify it
counter += 1 # Increment the global variable
def display():
local_var = "This is local" # Local variable
print(local_var)
print("Counter:", counter) # Accessing global variable
increment()
display()
# Trying to access local_var outside its scope will raise an error
# print(local_var) # Uncommenting this line will cause a NameError
NCERT Computer Science Class 11 Chapter 7 Solutions Q 6