Chapter #7 – Functions
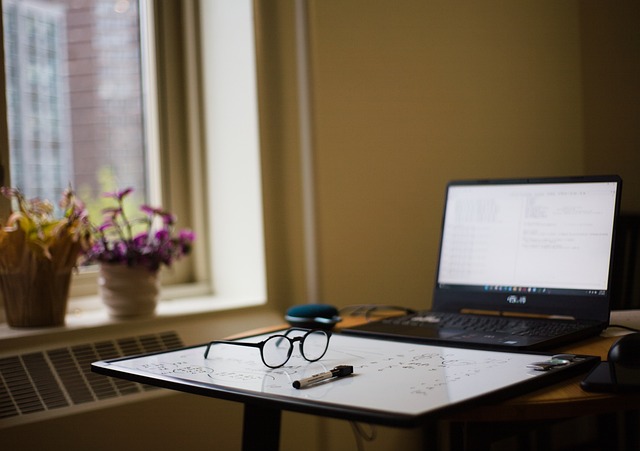
Chapter #7 – Lab Exercises #3
Write a program that has a user defined function to accept the coefficients of a quadratic equation in variables and calculates its determinant. For example : if the coefficients are stored in the variables a,b,c then calculate determinant as b2-4ac. Write the appropriate condition to check determinants on positive, zero and negative and output appropriate result.
def calculate_determinant(a, b, c):
"""Calculate the determinant of a quadratic equation."""
return b**2 - 4*a*c
def main():
# Accepting coefficients from the user
try:
a = float(input("Enter the coefficient a: "))
b = float(input("Enter the coefficient b: "))
c = float(input("Enter the coefficient c: "))
# Ensure 'a' is not zero for a valid quadratic equation
if a == 0:
print("Coefficient 'a' cannot be zero for a quadratic equation.")
return
# Calculate the determinant
determinant = calculate_determinant(a, b, c)
# Check the value of the determinant and output the result
if determinant > 0:
print("The determinant is positive. The quadratic equation has two distinct real roots.")
elif determinant == 0:
print("The determinant is zero. The quadratic equation has exactly one real root (repeated).")
else:
print("The determinant is negative. The quadratic equation has no real roots (two complex roots).")
except ValueError:
print("Invalid input. Please enter numeric values for coefficients.")
# Run the main function
if __name__ == "__main__":
main()
You can checkout the code from Github here – Lab Exercises #3
Explanation of the Code:
1. Function Definition:
• calculate_determinant(a, b, c): This function calculates the determinant
using the formula b2−4ac.
2. Main Function:
• Prompts the user to input the coefficients a, b, and c.
• Checks if aa is zero (since it would not be a quadratic equation).
• Calls calculate_determinant to compute the determinant.
• Evaluates the value of the determinant and prints messages based on whether
it is positive, zero, or negative.
Example 1: Positive Determinant:
Input:
Enter the coefficient a: 1
Enter the coefficient b: 5
Enter the coefficient c: 6
Output:
The determinant is positive. The quadratic equation has two distinct real roots.
Explanation:
The determinant calculated is 52 – 4 × 1 × 6 = 25 – 24 = 1 which is positive.
Example 2: Zero Determinant
Input:
Enter the coefficient a: 1
Enter the coefficient b: 4
Enter the coefficient c: 4
Output:
The determinant is zero. The quadratic equation has exactly one real root (repeated).
Explanation:
The determinant calculated is 42 – 4 × 1 × 4 = 16 – 16 = 0 which is zero.
Example 3: Negative Determinant
Input:
Enter the coefficient a: 1
Enter the coefficient b: 2
Enter the coefficient c: 5
Output:
The determinant is negative. The quadratic equation has no real roots (two complex roots).
Explanation:
The determinant calculated is 22 – 4 × 1 × 5 = 4 – 20 = −16 which is negative.
NCERT Computer Science Class 11 Chapter 7 Solutions Q 10