Chapter #7 – Functions
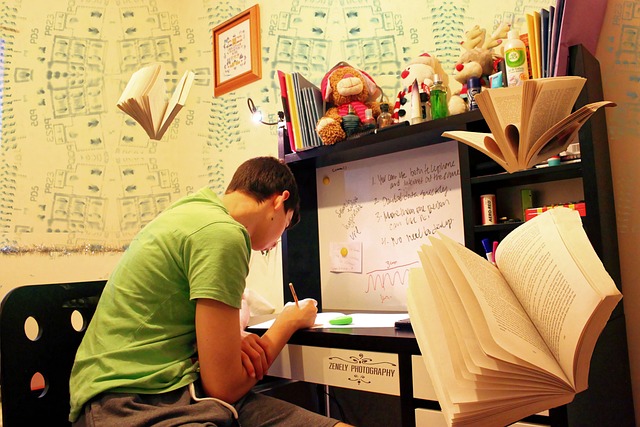
Chapter #7 – Lab Exercises #1
Write a program to check the divisibility of a number by 7 that is passed as a parameter to the user defined function.
def is_divisible_by_7(number):
"""
Check if the given number is divisible by 7.
Parameters:
number (int): The number to be checked.
Returns:
bool: True if the number is divisible by 7, False otherwise.
"""
return number % 7 == 0
# User input
try:
user_input = int(input("Enter a number to check its divisibility by 7: "))
# Check divisibility
if is_divisible_by_7(user_input):
print(f"{user_input} is divisible by 7.")
else:
print(f"{user_input} is not divisible by 7.")
except ValueError:
print("Please enter a valid integer.")
You can checkout this code from Github here – Lab Exercises – 1
How the Program Works:
Function Definition: The is_divisible_by_7 function checks if the input number is divisible by 7 using the modulus operator (%). If number % 7 equals zero, it returns True; otherwise, it returns False.
User Input: The program prompts the user to enter an integer. It uses a try-except block to handle any potential errors from invalid input (non-integer values).
Divisibility Check: The program calls the is_divisible_by_7 function with the user's input and prints whether the number is divisible by 7 or not.
Example Output
If the user enters 21, the output will be:
21 is divisible by 7.
If the user enters 10, the output will be:
10 is not divisible by 7.
NCERT Computer Science Class 11 Chapter 7 Solutions Q 8