Chapter #7 – Functions
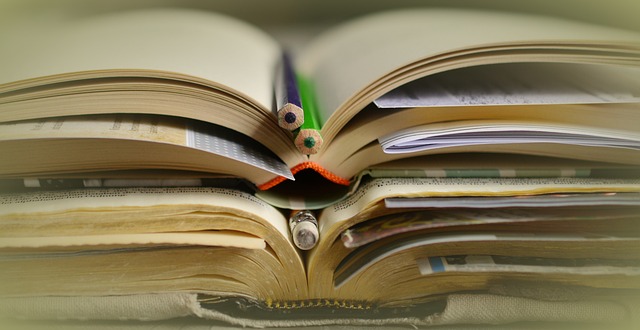
Chapter #7 – Q #7
Does a function always return a value? Explain with an example.
In Python, a function does not always return a value. If a function does not explicitly use a return statement, it will implicitly return None. This behavior allows functions to perform actions without necessarily providing a result.
Example of a Function Without a Return Value:
Consider the following function that prints a message but does not return any value:
def greet(name):
print(f"Hello, {name}!")
When you call this function:
result = greet("Alice")
print(result) # This will print: None
In this example, the greet function executes the print statement but does not return any value. Therefore, when you try to assign its output to result, it receives None, which is Python’s default return value when no return statement is present.
Example of a Function With a Return Value
Conversely, here’s an example of a function that returns a value:
def add(a, b):
return a + b
When you call this function:
sum_result = add(5, 3)
print(sum_result) # This will print: 8
In this case, the add function explicitly returns the sum of a and b, allowing the caller to use that returned value.
Conclusion
To summarize, while Python functions can return values using the return statement, they are not required to do so. If no return is specified, the function will implicitly return None. This flexibility allows for both action-oriented functions and those designed to compute and return values.
NCERT Computer Science Class 11 Chapter 7 Solutions Q 7