Chapter #6 – Flow of Control
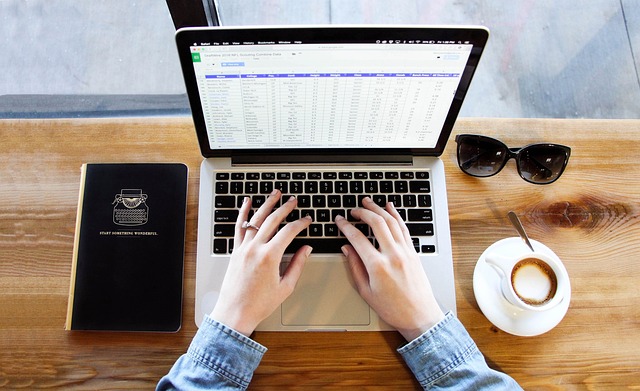
Class 11 – Chapter 6 Solutions – PROGRAMMING EXERCISES #10
Write a program to find the grade of a student when grades are allocated as given in the table below.
Percetage of Marks | Grade |
Above 90% | A |
80% to 90% | B |
70% to 80% | C |
60% to 70% | D |
Below 60% | E |
Percentage of the marks obtained by the student is input to the program.
Python Program:
def get_grade(percentage):
if percentage > 90:
return 'A'
elif 80 <= percentage <= 90:
return 'B'
elif 70 <= percentage < 80:
return 'C'
elif 60 <= percentage < 70:
return 'D'
else:
return 'E'
def main():
try:
# Input percentage from user
percentage = float(input("Enter the percentage of marks obtained by the student: "))
# Validate input
if percentage < 0 or percentage > 100:
print("Please enter a valid percentage between 0 and 100.")
return
# Get the grade
grade = get_grade(percentage)
# Output the grade
print(f"The grade for the student with {percentage}% marks is: {grade}")
except ValueError:
print("Invalid input! Please enter a numeric value for the percentage.")
if __name__ == "__main__":
main()
Checkout this code from Github here – Programming Exercises – 10
Example Output:
Enter the percentage of marks obtained by the student: 91
The grade for the student with 91.0% marks is: A
Enter the percentage of marks obtained by the student: 85
The grade for the student with 85.0% marks is: B
Enter the percentage of marks obtained by the student: 75
The grade for the student with 75.0% marks is: C
Enter the percentage of marks obtained by the student: 65
The grade for the student with 65.0% marks is: D
Enter the percentage of marks obtained by the student: 55
The grade for the student with 55.0% marks is: E
NCERT Computer Science Class 11 Chapter 6 Solutions Q 15