Chapter #6 – Flow of Control
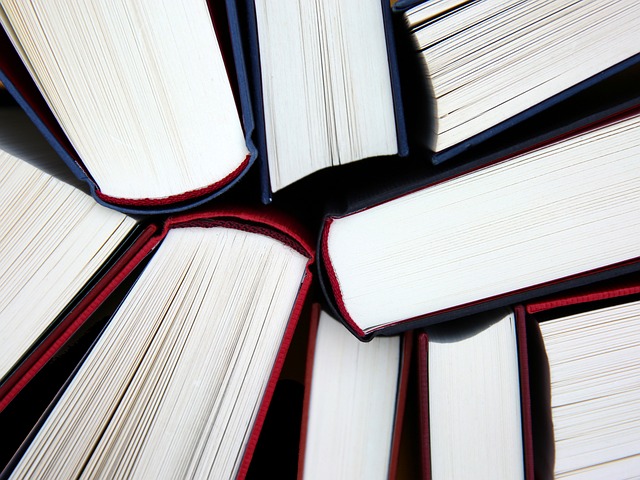
Class 11 – Chapter 6 Solutions – PROGRAMMING EXERCISES #9
(i) Write a program to print the following patterns:
*
* * *
* * * * *
* * *
*
Python Program:
def print_pattern():
# Define the number of rows for the upper part of the pattern
rows = 3
# Upper part of the pattern
for i in range(rows):
# Print leading spaces
print(" " * (rows - i - 1), end="")
# Print stars with spaces
if i == 2: # For the third row, print 4 stars
print("* " * 4)
else:
print("* " * (2 * i + 1))
# Lower part of the pattern
for i in range(rows - 1):
# Print leading spaces
print(" " * (i + 1), end="")
# Print stars with spaces
print("* " * (2 * (rows - i - 1) - 1))
# Call the function to print the pattern
print_pattern()
(ii) Write a program to generate below pattern:
1
2 1 2
3 2 1 2 3
4 3 2 1 2 3 4
5 4 3 2 1 2 3 4 5
Python Program:
def print_pattern(n):
for i in range(1, n + 1):
# Print leading spaces
print(" " * (n - i) * 2, end="") # Multiply by 2 for better alignment
# Print descending numbers
for j in range(i, 0, -1):
print(j, end=" ")
# Print ascending numbers
for j in range(2, i + 1):
print(j, end=" ")
# Move to the next line after each row
print()
# Set the number of rows for the pattern
rows = 5
print_pattern(rows)
(iii) Write a program to generate below pattern:
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Python Program:
def print_pattern(n):
for i in range(n):
# Print leading spaces
print(" " * (2 * i), end="") # Multiply by 2 for better alignment
# Print numbers from 1 to n-i
for j in range(1, n - i + 1):
print(j, end=" ")
# Move to the next line after each row
print()
# Set the number of rows for the pattern
rows = 5
print_pattern(rows)
NCERT Computer Science Class 11 Chapter 6 Solutions Q 14